Visual C++ extends the ANSI C and ANSI C++ standards as follows.
Extension for Visual Studio Code - C/C++ IntelliSense, debugging, and code browsing. Visual studio for mac tfs rivkadehan reported Oct 28 at 03:22 PM I have installed visual studio for mac and cannot install TFS Extension. Visual Studio for Mac enables the creation of.NET Core solutions, providing the back-end services to your client solutions. Code, debug, and test these cloud services simultaneously with your client solutions for increased productivity.
Visual Studio Code is a completely different (and much less advanced) tool than the standard Visual Studio product line. VS Code is more similar to Atom or Sublime. But it does seem to be improving very quickly. There has no option to create C++ project in this version and some other community members reported this suggestion to the Visual Studio Product Team, please check this: Support C++ in Visual Studio for Mac and you can vote it, then waiting for the feedback from the Visual Studio Product Team. Visual Studio 2017 for Mac is actually a different beast from Visual Studio for Windows. As you may know, it is originally based on Xamarin Studio which on its turn is an extended form of MonoDevelop. Download C# IL Viewer for Visual Studio Code or install it directly within Visual Studio Code by launching Quick Open (CMD+P for Mac or CTRL+P for Windows) and pasting in the follow command and press enter.
Keywords
Several keywords are added. In the list in Keywords, the keywords that have two leading underscores are Visual C++ extensions.
Out of class definition of static const integral (or enum) members
Under the standard (/Za), you must make an out-of-class definition for data members, as shown here:
Visual Studio For Mac C++ Extension
Under /Ze, the out-of-class definition is optional for static, const integral, and const enum data members. Only integrals and enums that are static and const can have initializers in a class; the initializing expression must be a const expression.
To avoid errors when an out-of-class definition is provided in a header file and the header file is included in multiple source files, use selectany. For example:
Casts
Both the C++ compiler and C compiler support these kinds of non-ANSI casts:
Non-ANSI casts to produce l-values. For example:
Note
This extension is available in the C language only. You can use the following ANSI C standard form in C++ code to modify a pointer as if it is a pointer to a different type.
The preceding example could be rewritten as follows to conform to the ANSI C standard.
Non-ANSI casts of a function pointer to a data pointer. For example:
To perform the same cast and also maintain ANSI compatibility, you can cast the function pointer to a
uintptr_t
before you cast it to a data pointer:
Variable-length argument lists
Both the C++ compiler and C compiler support a function declarator that specifies a variable number of arguments, followed by a function definition that provides a type instead:
Single-line comments
The C compiler supports single-line comments, which are introduced by using two forward slash (//) characters:
Scope
The C compiler supports the following scope-related features.
Visual Studio For Mac Extensions
Redefinitions of extern items as static:
Use of benign typedef redefinitions within the same scope:
Function declarators have file scope:
Use of block-scope variables that are initialized by using nonconstant expressions:
Data declarations and definitions
The C compiler supports the following data declaration and definition features.
Mixed character and string constants in an initializer:
Bit fields that have base types other than unsigned int or signed int.
Declarators that don't have a type:
Unsized arrays as the last field in structures and unions:
Unnamed (anonymous) structures:
Unnamed (anonymous) unions:
Unnamed members:
Intrinsic floating-point functions
Both the x86 C++ compiler and C compiler support inline generation of the atan
, atan2
, cos
, exp
, log
, log10
, sin
, sqrt
, and tan
functions when /Oi is specified. For the C compiler, ANSI conformance is lost when these intrinsics are used, because they do not set the errno
variable.
Passing a non-const pointer parameter to a function that expects a reference to a const pointer parameter
This is an extension to C++. This code will compile with /Ze:
ISO646.H not enabled
Under /Ze, you have to include iso646.h if you want to use text forms of the following operators:
&& (and)
&= (and_eq)
& (bitand)
| (bitor)
~ (compl)
! (not)
!= (not_eq)
|| (or)
|= (or_eq)
^ (xor)
^= (xor_eq)
Address of string literal has type const char [], not const char (*) []
The following example will output char const (*)[4]
under /Za, but char const [4]
under /Ze.
See also
I've begun using VSC for my embedded C projects with gcc for ARM on a Mac. Having set up include paths in c_cpp_properties.json
, most of my #includes
are now working. However, a line such as this:
produces a red squiggly underline and the error:
The source file in question includes stdint:
and the includePath
includes:
and:
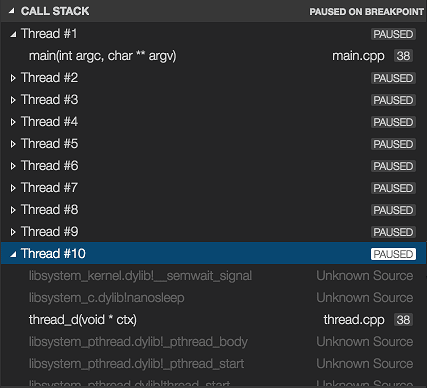
(the only other option being msvc-x64
).
The codebase compiles just fine when I use make and gcc. How do I show the C/C++ extension where uint32_t
is?
Edit:
stdint.h
looks like this:

and stdint-gcc.h
contains:
This suggests __UINT32_TYPE__
is NOT defined when VSC is parsing my code, but it IS defined when I build with make and gcc.
Edit:
Following @mbmcavoy's answer I'm including my c_cpp_properties.json
file here:
Edit:
On digging deeper, I found that gcc-arm-none-eabi-4_9-2015q3/lib/gcc/arm-none-eabi/4.9.3/include/stdint.h
had __STDC_HOSTED__
defined and therefore stdint-gcc.h
was not actually being included. Instead, that header does an 'include_next <stdint.h>
', which finds gcc-arm-none-eabi-4_9-2015q3/arm-none-eabi/include/stdint.h
. I still can't see where unint32_t is defined, either for gcc and make or for VSC.
2 Answers
After trying all of the proposed solutions to no effect, I consider the uint32_t issue to be a bug.
To solve the annoying warnings in VSCode, just add the following line after your #include section:
By doing this once in a single file, it fixes my VSCode warnings and still compiles.
wscourgeI have been able to resolve this on my machine (Windows), with three steps:
- Applying Compiler Defines to the C/C++ Extension
The question is correct when it states 'This suggests __UINT32_TYPE__
is NOT defined when VSC is parsing my code, but it IS defined when I build with make and gcc.' The ARM cross-compiler has many built-in defines that are not included in the clang-x64 parser.
First, find out defines your gcc compiler defines, with the -dM -E
options. On Windows I was able to dump the output to a file with echo | arm-none-eabi-gcc -dM -E - > gcc-defines.txt
Second, add the defines to your c_cpp_properties.json
file. Note that where the #define sets a value, you need to use an =
sign here. (You could probably just add individual defines as you need them, but I used Excel to format them as needed and sort. The first defines are for my project, matching the defines in my Makefile.)
- Setting the Symbol Database
After doing a few experiments with individual defines, I could see the define as being processed in stdint-gcc.h
, any uses of the types still produced errors. I realized in my c_cpp_properties.json
file that I had 'databaseFilename': '
This is used for the 'generated symbol database', but was not configured properly. I set it to:
- Restart Visual Studio Code
After quitting and restarting Visual Studio Code, declarations do not result in the error, and when hovering over a variable, it shows the appropriate type.
mbmcavoymbmcavoy